Default WordPress Image Sizes
WordPress supports several image sizes by default. You can change them in admin area: Settings > Media
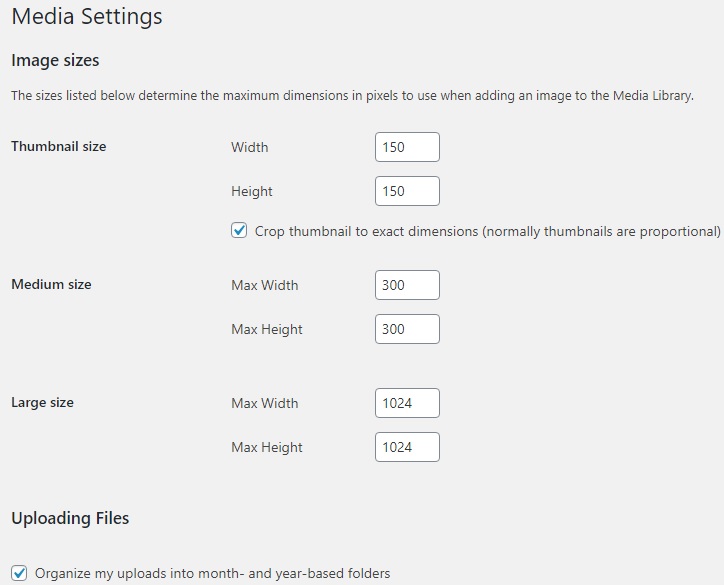
A better way to do it is from functions.php
of your theme. Add this hook filter:
add_filter('image_size_names_choose', 'image_size_names');
function image_size_names($sizes) {
var_dump($sizes); die;
}
Then, from your admin dashboard, add a new post /wp-admin/post-new.php
. Now you can see the default image sizes:
G:\your_website\wp-content\themes\your_theme\functions.php:
array (size=4)
'thumbnail' => string 'Thumbnail' (length=9)
'medium' => string 'Medium' (length=6)
'large' => string 'Large' (length=5)
'full' => string 'Full Size' (length=9)
Add New Image Sizes
Let’s say you want to “update” the existing sizes and add 2 new ones: ‘small’ and ‘medium_large’.
function remove_default_sizes() {
remove_image_size('thumbnail');
remove_image_size('medium');
remove_image_size('large');
remove_image_size('full');
}
function custom_image_sizes() {
remove_default_sizes();
// new image: name, width, height (auto)
add_image_size( 'thumbnail', 300, 9999 );
add_image_size( 'small', 350, 9999 );
add_image_size( 'medium', 400, 9999 );
add_image_size( 'large', 500, 9999 );
add_image_size( 'medium_large', 600, 9999 );
add_image_size( 'full', 700, 9900 );
}
add_action('after_setup_theme', 'custom_image_sizes');
function image_size_names($sizes) {
return array_merge( $sizes, array(
'thumbnail' => __( 'Thumbnail' ),
'small' => __( 'Small' ),
'medium' => __( 'Medium' ),
'medium_large' => __( 'Medium Large' ),
'large' => __( 'Large' ),
'full' => __( 'Full' )
));
}
add_filter('image_size_names_choose', 'image_size_names');
Now, if you upload a picture in your article (post), let’s say: building.jpg with 3264 x 1836 px, WordPress will generate all sizes:
<figure class="wp-block-image size-large"> <img src="http://website/building-500x281.jpg" alt="" class="wp-image-464" srcset=" http://website/building-500x281.jpg 500w, http://website/building-400x225.jpg 400w, http://website/building-300x169.jpg 300w, http://website/building-600x338.jpg 600w, http://website/building-350x197.jpg 350w, http://website/building-700x394.jpg 700w " sizes="(max-width: 500px) 100vw, 500px" /> </figure>
Change the default image media queries
By default, WordPress creates ony one media query. In our example, sizes="(max-width: 500px) 100vw, 500px"
which means that for screen sizes smaller than 500px the picture will have 100% width of view-port otherwise it will be 500px.
The break-point of 500px can be changed by updating the image or figure class with ‘size-{image-size-name}’.
Let’s make each image size width a breakpoint:
1. In admin, change the article image option to ‘Full Size’
<figure class="wp-block-image size-full"> <img src="http://website/building-700x394.jpg" class="wp-image-464"/> </figure>
2. Add this filter in functions.php
function responsive_image_sizes($sizes, $size) {
global $_wp_additional_image_sizes;
$width = $size[0];
$list = array_values($_wp_additional_image_sizes);
$len = count($list);
$res = '';
for($i=1; $i<$len; $i++) {
$res .= '(max-width: '. $list[$i]['width'].'px) '. $list[$i-1]['width'].'px, ';
}
$res .= $width.'px';
return $res;
}
add_filter('wp_calculate_image_sizes', 'responsive_image_sizes', 10 , 2);
Now the page will have the html like:
<figure class="wp-block-image size-full"> <img src="http://website/building-700x394.jpg" alt="" class="wp-image-464" srcset=" http://website/building-700x394.jpg 700w, http://website/building-400x225.jpg 400w, http://website/building-500x281.jpg 500w, http://website/building-300x169.jpg 300w, http://website/building-600x338.jpg 600w, http://website/building-350x197.jpg 350w " sizes="(max-width: 350px) 300px, (max-width: 400px) 350px, (max-width: 500px) 400px, (max-width: 600px) 500px, (max-width: 700px) 600px, 700px" /> </figure>
What about Featured Images ?
It will become responsive also, like all the other images in the page. If you need to keep them non-responsive (at the original size), add this filter in functions.php
function update_image_attr($attr, $post, $size='full') {
unset($attr['srcset']);
unset($attr['sizes']);
$attr['class'] = '';
return $attr;
}
add_filter('wp_get_attachment_image_attributes', 'update_image_attr', 10, 3);
That's all, now you're enough 'responsive' 🙂
not enough for so many screen sizes:)